Documentation 12
// Final Project Idea
So I reframe the project’s idea from a 24-hour clock to a minute timer while asking the participants to view their heartbeat, counting 60 seconds when having their hands on the sensor. The hands on one side of the timer will go according to the participants’ heartbeat in a loop. After they done counting, they will release their hands from the sensor and mark a dot on the tracing paper attached to the clock surface.
I did 2 testings using the Serial plotter of the heartbeat. One of the responses I received is that the environment’s sound really distracted them from focusing on counting.
video 12.1 testing 1
video 12.2 testing 2
I then started with programing the arduino to let it light up the LED whenever it receives a pulse. I did it by comparing the first, the second and the third IR value got from the sensor. Initially I tried to do with arrays, yet I didn’t proceed with this, for I did not know the length of the array. Thus I put them in a while loop as an alternative. I also first tried with seeing what will happen with the IR values’ change by printing out words to test out.
Here is the test code:
Part of the test code:
int pulse = particleSensor.getIR();
//Send raw data to plotter
if (pulse > 120000){
Serial.println("HI");
}else{
Serial.println("NO");
}
int pulse = particleSensor.getIR();
//Send raw data to plotter
if (pulse > 120000){
Serial.println("HI");
}else{
Serial.println("NO");
}
Code lighting up LED and moving the stepper motor according to each peak of the pulse:#include <Stepper.h>
#include <Wire.h>
#include "MAX30105.h"
MAX30105 particleSensor;
const int ledWhite = 2;
const int stepsPerRevolution = 60;
Stepper myStepper(stepsPerRevolution, 8,9,10,11);
// initialize the stepper library on pins 8 through 11:
void setup(){
Serial.begin(115200); Serial.println("Initializing...");
pinMode(ledWhite, OUTPUT);
// Initialize sensor
if (!particleSensor.begin(Wire, I2C_SPEED_FAST)) //Use default I2C port, 400kHz speed { Serial.println("MAX30105 was not found. Please check wiring/power. ");
while (1);
}
//Setup to sense a nice looking saw tooth on the plotter byte ledBrightness = 0x1F;
//Options: 0=Off to 255=50mA
byte sampleAverage = 8;
//Options: 1, 2, 4, 8, 16, 32
byte ledMode = 3;
//Options: 1 = Red only, 2 = Red + IR, 3 = Red + IR + Green
int sampleRate = 100;
//Options: 50, 100, 200, 400, 800, 1000, 1600, 3200
int pulseWidth = 411;
//Options: 69, 118, 215, 411
int adcRange = 4096;
//Options: 2048, 4096, 8192, 16384
particleSensor.setup(ledBrightness, sampleAverage, ledMode, sampleRate, pulseWidth, adcRange);
//Configure sensor with these settings
}
void loop(){
int pulse;
while((pulse = particleSensor.getIR()) != 0){
int pulse2 = particleSensor.getIR();
int pulse3 = particleSensor.getIR();
if ((pulse3<pulse2) && (pulse2>pulse) && pulse2 !=0 && pulse3 != 0 && pulse2 > 100000 && pulse3 > 100000){
digitalWrite(ledWhite,HIGH);
myStepper.step(5);
Serial.println(pulse2);
}else{ digitalWrite(ledWhite,LOW);
}
}
}
#include <Wire.h>
#include "MAX30105.h"
MAX30105 particleSensor;
const int ledWhite = 2;
const int stepsPerRevolution = 60;
Stepper myStepper(stepsPerRevolution, 8,9,10,11);
// initialize the stepper library on pins 8 through 11:
void setup(){
Serial.begin(115200); Serial.println("Initializing...");
pinMode(ledWhite, OUTPUT);
// Initialize sensor
if (!particleSensor.begin(Wire, I2C_SPEED_FAST)) //Use default I2C port, 400kHz speed { Serial.println("MAX30105 was not found. Please check wiring/power. ");
while (1);
}
//Setup to sense a nice looking saw tooth on the plotter byte ledBrightness = 0x1F;
//Options: 0=Off to 255=50mA
byte sampleAverage = 8;
//Options: 1, 2, 4, 8, 16, 32
byte ledMode = 3;
//Options: 1 = Red only, 2 = Red + IR, 3 = Red + IR + Green
int sampleRate = 100;
//Options: 50, 100, 200, 400, 800, 1000, 1600, 3200
int pulseWidth = 411;
//Options: 69, 118, 215, 411
int adcRange = 4096;
//Options: 2048, 4096, 8192, 16384
particleSensor.setup(ledBrightness, sampleAverage, ledMode, sampleRate, pulseWidth, adcRange);
//Configure sensor with these settings
}
void loop(){
int pulse;
while((pulse = particleSensor.getIR()) != 0){
int pulse2 = particleSensor.getIR();
int pulse3 = particleSensor.getIR();
if ((pulse3<pulse2) && (pulse2>pulse) && pulse2 !=0 && pulse3 != 0 && pulse2 > 100000 && pulse3 > 100000){
digitalWrite(ledWhite,HIGH);
myStepper.step(5);
Serial.println(pulse2);
}else{ digitalWrite(ledWhite,LOW);
}
}
}
Here is the result I got. However, I don’t think it is getting every peak of the pulses, or there is some non-detected pulses. How can I fix this issue?
video 12.3 connecting the circuit
SiI then did some sketches of the fabrication. Here is a very rough draft of how everything will be contained. For the final result, I am thinking of soldering the sensor so it can be attached somewhere outside the breadboard. Right now its position is a bit awkward.
![]()
video 12.4 paper prototype
Question:
Can I include 2 stepper libraries inside the code? I am imagining the stepper motors’ hand will be reset to its original position after 1 participant finished doing the interaction. Yet, to do that, I will need to include another library called AccelStepper which has functions like stepper.moveTo(), which is not included in the standard stepper library.
Resources:
resetting the stepper motor’s position: https://community.element14.com/members-area/personalblogs/b/blog/posts/arduino-controlling-stepper-motor-28byj-48-with-accelstepper-library
video 12.3 connecting the circuit
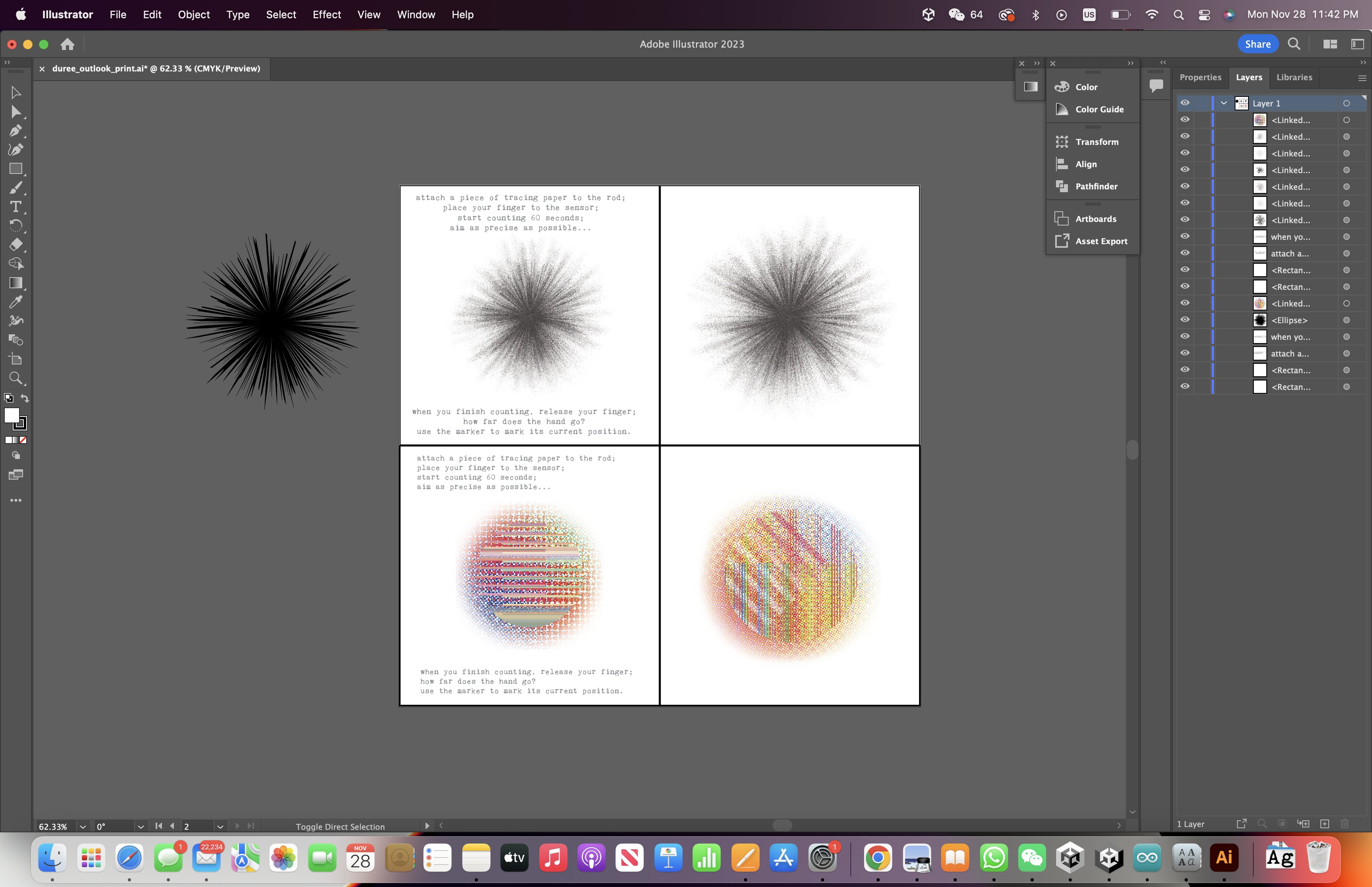
video 12.4 paper prototype
Question:
Can I include 2 stepper libraries inside the code? I am imagining the stepper motors’ hand will be reset to its original position after 1 participant finished doing the interaction. Yet, to do that, I will need to include another library called AccelStepper which has functions like stepper.moveTo(), which is not included in the standard stepper library.
Resources:
resetting the stepper motor’s position: https://community.element14.com/members-area/personalblogs/b/blog/posts/arduino-controlling-stepper-motor-28byj-48-with-accelstepper-library